mirror of
https://github.com/konvajs/konva.git
synced 2025-07-15 11:24:49 +08:00
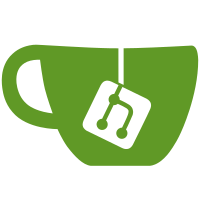
I added the experimental folder to show some work on filters that can be applied to an entire layer. Multiple filters can be applied to a layer (in any order, multiple times). To hook into the layer I use: layer.on('draw', filterFunc); Eventually, I would like to move that to `layer.filterFunc` and automatically apply it after the draw. `filterFunc` looks like: function filterFunc(){ // Get pixel data and create a temporary pixel buffer for working var imageData = this.getContext().getImageData(0,0,this.getCanvas().width,this.getCanvas().height); var scratchData = this.getContext().createImageData(imageData); // Apply all filters here ColorStretch(imageData,scratchData,{}); // Copy the pixel data back this.getContext().putImageData(scratchData,0,0); } `ColorStretch` is an example of a filter. It takes 3 arguments: the original image data, image data to write the result to, and an options object.
42 lines
1.2 KiB
JavaScript
42 lines
1.2 KiB
JavaScript
FlipX = (function () {
|
|
var FlipX = function (src, dst, opt) {
|
|
var srcPixels = src.data,
|
|
dstPixels = dst.data,
|
|
xSize = src.width,
|
|
ySize = src.height,
|
|
i, m, x, y;
|
|
for (x = 0; x < xSize; x += 1) {
|
|
for (y = 0; y < ySize; y += 1) {
|
|
i = (y * xSize + x) * 4; // original
|
|
m = ((y + 1) * xSize - x) * 4; // flipped
|
|
dstPixels[m + 0] = srcPixels[i + 0];
|
|
dstPixels[m + 1] = srcPixels[i + 1];
|
|
dstPixels[m + 2] = srcPixels[i + 2];
|
|
dstPixels[m + 3] = srcPixels[i + 3];
|
|
}
|
|
}
|
|
};
|
|
return FlipX;
|
|
})();
|
|
|
|
FlipY = (function () {
|
|
var FlipY = function (src, dst, opt) {
|
|
var srcPixels = src.data,
|
|
dstPixels = dst.data,
|
|
xSize = src.width,
|
|
ySize = src.height,
|
|
i, m, x, y;
|
|
for (x = 0; x < xSize; x += 1) {
|
|
for (y = 0; y < ySize; y += 1) {
|
|
i = (y * xSize + x) * 4; // original
|
|
m = ((ySize - y) * xSize + x) * 4; // flipped
|
|
dstPixels[m + 0] = srcPixels[i + 0];
|
|
dstPixels[m + 1] = srcPixels[i + 1];
|
|
dstPixels[m + 2] = srcPixels[i + 2];
|
|
dstPixels[m + 3] = srcPixels[i + 3];
|
|
}
|
|
}
|
|
};
|
|
return FlipY;
|
|
})();
|