mirror of
https://github.com/OrchardCMS/Orchard.git
synced 2025-04-05 19:36:23 +08:00
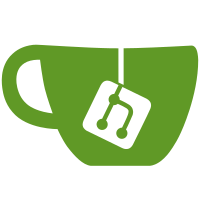
* 6748: Stricter file and folder name validation (#6792) * Media Library: More strict file and folder name validation, fixes #6748 * Resetting MediaLibraryService changes to 1.10.x * Code styling in FileSystemStorageProvider * Adding string file and folder name validation to FileSystemStorageProvider, so that MediaLibrary components don't need to do it separately * Applying the same file and folder name validation to AzureFileSystem too * Code styling and fixes in AzureFileSystem, MediaLibrary and IStorageProvider * Simplifying invalid character detection * Code styling * Adding InvalidNameCharacterException to be able to handle invalid characters precisely at various user-facing components * Updating MediaLibrary not to log an error when a file can't be uploaded due to invalid characters --------- Co-authored-by: Lombiq <github@lombiq.com> * #6793: Adding a content-independent culture selector shape for the front-end (#8784) * Adds a new CultureSelector shape for front-end * fixed query string culture change * Moving NameValueCollectionExtensions from Orchard.DynamicForms and Orchard.Localization to Orchard.Framework * Code styling * Simplifying UserCultureSelectorController and removing the addition of the culture to the query string * EOF empty lines and code styling * Fixing that the main Orchard.Localization should depend on Orchard.Autoroute * Code styling in LocalizationService * Updating LocalizationService to not have to use IEnumerable.Single * Matching culture name matching in LocalizationService culture- and casing-invariant --------- Co-authored-by: Sergio Navarro <jersio@hotmail.com> Co-authored-by: psp589 <pablosanchez589@gmail.com> * #8640: Fixing consistency between different Enumeration Field flavors' data storage (#8789) * Reworking EnumerationField's logic to store/retrieve its (selected) values * Fixing exception when creating new item with CheckboxList flavor, adding more nullchecks and compactness * Code styling in EnumerationFieldDriver * Code styling in EnumerationField editor template * Fixing that EnumerationFieldDriver and the EnumerationField editor template should read SelectedValues instead of Values directly --------- Co-authored-by: Matteo Piovanelli <MatteoPiovanelli-Laser@users.noreply.github.com> * Fixing merge --------- Co-authored-by: Lombiq <github@lombiq.com> Co-authored-by: Sergio Navarro <jersio@hotmail.com> Co-authored-by: psp589 <pablosanchez589@gmail.com> Co-authored-by: Matteo Piovanelli <MatteoPiovanelli-Laser@users.noreply.github.com>
86 lines
3.9 KiB
C#
86 lines
3.9 KiB
C#
using System;
|
|
using Orchard.ContentManagement;
|
|
using Orchard.ContentManagement.Drivers;
|
|
using Orchard.FileSystems.Media;
|
|
using Orchard.Localization;
|
|
using Orchard.MediaLibrary.Models;
|
|
using Orchard.MediaLibrary.Services;
|
|
using Orchard.Security;
|
|
using Orchard.UI.Notify;
|
|
|
|
namespace Orchard.MediaLibrary.MediaFileName {
|
|
public class MediaFileNameDriver : ContentPartDriver<MediaPart> {
|
|
private readonly IAuthenticationService _authenticationService;
|
|
private readonly IAuthorizationService _authorizationService;
|
|
private readonly IMediaLibraryService _mediaLibraryService;
|
|
private readonly INotifier _notifier;
|
|
|
|
public MediaFileNameDriver(IAuthorizationService authorizationService, IAuthenticationService authenticationService, IMediaLibraryService mediaLibraryService, INotifier notifier) {
|
|
_authorizationService = authorizationService;
|
|
_authenticationService = authenticationService;
|
|
_mediaLibraryService = mediaLibraryService;
|
|
_notifier = notifier;
|
|
|
|
T = NullLocalizer.Instance;
|
|
}
|
|
|
|
public Localizer T { get; set; }
|
|
|
|
protected override string Prefix {
|
|
get { return "MediaFileName"; }
|
|
}
|
|
|
|
protected override DriverResult Editor(MediaPart part, dynamic shapeHelper) {
|
|
return Editor(part, null, shapeHelper);
|
|
}
|
|
|
|
protected override DriverResult Editor(MediaPart part, IUpdateModel updater, dynamic shapeHelper) {
|
|
return ContentShape(
|
|
"Parts_Media_Edit_FileName",
|
|
() => {
|
|
var currentUser = _authenticationService.GetAuthenticatedUser();
|
|
if (!_authorizationService.TryCheckAccess(Permissions.EditMediaContent, currentUser, part)) {
|
|
return null;
|
|
}
|
|
|
|
var settings = part.TypeDefinition.Settings.GetModel<MediaFileNameEditorSettings>();
|
|
if (!settings.ShowFileNameEditor) {
|
|
return null;
|
|
}
|
|
|
|
MediaFileNameEditorViewModel model = shapeHelper.Parts_Media_Edit_FileName(typeof(MediaFileNameEditorViewModel));
|
|
|
|
if (part.FileName != null) {
|
|
model.FileName = part.FileName;
|
|
}
|
|
|
|
if (updater != null) {
|
|
var priorFileName = model.FileName;
|
|
if (updater.TryUpdateModel(model, Prefix, null, null)) {
|
|
if (model.FileName != null && !model.FileName.Equals(priorFileName, StringComparison.OrdinalIgnoreCase)) {
|
|
var fieldName = "MediaFileNameEditorSettings.FileName";
|
|
|
|
try {
|
|
_mediaLibraryService.RenameFile(part.FolderPath, priorFileName, model.FileName);
|
|
part.FileName = model.FileName;
|
|
|
|
_notifier.Add(NotifyType.Success, T("File '{0}' was renamed to '{1}'", priorFileName, model.FileName));
|
|
}
|
|
catch (OrchardException) {
|
|
updater.AddModelError(fieldName, T("Unable to rename file. Invalid Windows file path."));
|
|
}
|
|
catch (InvalidNameCharacterException) {
|
|
updater.AddModelError(fieldName, T("The file name contains invalid character(s)."));
|
|
}
|
|
catch (Exception exception) {
|
|
updater.AddModelError(fieldName, T("Unable to rename file: {0}", exception.Message));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
return model;
|
|
});
|
|
}
|
|
}
|
|
} |