mirror of
https://github.com/OrchardCMS/Orchard.git
synced 2025-04-05 19:36:23 +08:00
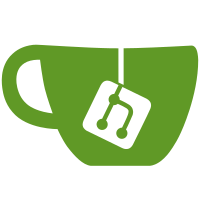
* 6748: Stricter file and folder name validation (#6792) * Media Library: More strict file and folder name validation, fixes #6748 * Resetting MediaLibraryService changes to 1.10.x * Code styling in FileSystemStorageProvider * Adding string file and folder name validation to FileSystemStorageProvider, so that MediaLibrary components don't need to do it separately * Applying the same file and folder name validation to AzureFileSystem too * Code styling and fixes in AzureFileSystem, MediaLibrary and IStorageProvider * Simplifying invalid character detection * Code styling * Adding InvalidNameCharacterException to be able to handle invalid characters precisely at various user-facing components * Updating MediaLibrary not to log an error when a file can't be uploaded due to invalid characters --------- Co-authored-by: Lombiq <github@lombiq.com> * #6793: Adding a content-independent culture selector shape for the front-end (#8784) * Adds a new CultureSelector shape for front-end * fixed query string culture change * Moving NameValueCollectionExtensions from Orchard.DynamicForms and Orchard.Localization to Orchard.Framework * Code styling * Simplifying UserCultureSelectorController and removing the addition of the culture to the query string * EOF empty lines and code styling * Fixing that the main Orchard.Localization should depend on Orchard.Autoroute * Code styling in LocalizationService * Updating LocalizationService to not have to use IEnumerable.Single * Matching culture name matching in LocalizationService culture- and casing-invariant --------- Co-authored-by: Sergio Navarro <jersio@hotmail.com> Co-authored-by: psp589 <pablosanchez589@gmail.com> * #8640: Fixing consistency between different Enumeration Field flavors' data storage (#8789) * Reworking EnumerationField's logic to store/retrieve its (selected) values * Fixing exception when creating new item with CheckboxList flavor, adding more nullchecks and compactness * Code styling in EnumerationFieldDriver * Code styling in EnumerationField editor template * Fixing that EnumerationFieldDriver and the EnumerationField editor template should read SelectedValues instead of Values directly --------- Co-authored-by: Matteo Piovanelli <MatteoPiovanelli-Laser@users.noreply.github.com> * Fixing merge --------- Co-authored-by: Lombiq <github@lombiq.com> Co-authored-by: Sergio Navarro <jersio@hotmail.com> Co-authored-by: psp589 <pablosanchez589@gmail.com> Co-authored-by: Matteo Piovanelli <MatteoPiovanelli-Laser@users.noreply.github.com>
76 lines
2.7 KiB
C#
76 lines
2.7 KiB
C#
using System.Web;
|
|
using Orchard.Environment.Configuration;
|
|
using Orchard.Environment.Extensions;
|
|
using Orchard.Localization.Providers;
|
|
using Orchard.Localization.Services;
|
|
using Orchard.Mvc;
|
|
using Orchard.Services;
|
|
using Orchard.UI.Admin;
|
|
|
|
namespace Orchard.Localization.Selectors {
|
|
[OrchardFeature("Orchard.Localization.CultureSelector")]
|
|
public class CookieCultureSelector : ICultureSelector, ICultureStorageProvider {
|
|
private readonly IHttpContextAccessor _httpContextAccessor;
|
|
private readonly IClock _clock;
|
|
private readonly ShellSettings _shellSettings;
|
|
|
|
private const string FrontEndCookieName = "OrchardCurrentCulture-FrontEnd";
|
|
private const string AdminCookieName = "OrchardCurrentCulture-Admin";
|
|
private const int DefaultExpireTimeYear = 1;
|
|
|
|
public CookieCultureSelector(
|
|
IHttpContextAccessor httpContextAccessor,
|
|
IClock clock,
|
|
ShellSettings shellSettings) {
|
|
_httpContextAccessor = httpContextAccessor;
|
|
_clock = clock;
|
|
_shellSettings = shellSettings;
|
|
}
|
|
|
|
public void SetCulture(string culture) {
|
|
var httpContext = _httpContextAccessor.Current();
|
|
|
|
if (httpContext == null) return;
|
|
|
|
var cookieName = AdminFilter.IsApplied(httpContext) ? AdminCookieName : FrontEndCookieName;
|
|
|
|
var cookie = new HttpCookie(cookieName, culture) {
|
|
Expires = _clock.UtcNow.AddYears(DefaultExpireTimeYear),
|
|
Domain = httpContext.Request.IsLocal ? null : httpContext.Request.Url.Host
|
|
};
|
|
|
|
if (!string.IsNullOrEmpty(_shellSettings.RequestUrlPrefix)) {
|
|
cookie.Path = GetCookiePath(httpContext);
|
|
}
|
|
|
|
httpContext.Request.Cookies.Remove(cookieName);
|
|
httpContext.Response.Cookies.Remove(cookieName);
|
|
httpContext.Response.Cookies.Add(cookie);
|
|
}
|
|
|
|
public CultureSelectorResult GetCulture(HttpContextBase context) {
|
|
if (context == null) return null;
|
|
|
|
var cookieName = AdminFilter.IsApplied(context) ? AdminCookieName : FrontEndCookieName;
|
|
|
|
var cookie = context.Request.Cookies.Get(cookieName);
|
|
|
|
if (cookie != null)
|
|
return new CultureSelectorResult { Priority = -1, CultureName = cookie.Value };
|
|
|
|
return null;
|
|
}
|
|
|
|
private string GetCookiePath(HttpContextBase httpContext) {
|
|
var cookiePath = httpContext.Request.ApplicationPath;
|
|
if (cookiePath != null && cookiePath.Length > 1) {
|
|
cookiePath += '/';
|
|
}
|
|
|
|
cookiePath += _shellSettings.RequestUrlPrefix;
|
|
|
|
return cookiePath;
|
|
}
|
|
}
|
|
}
|